The modern software development landscape is characterized by its rapid pace and constant evolution. To keep up with the demands of the market, developers need efficient, automated, and reliable practices. Full-stack applications, which encompass both front-end and back-end components, are becoming increasingly prevalent. Developing these applications efficiently and ensuring a smooth deployment process are challenges that every development team faces. Continuous Integration and Continuous Deployment (CI/CD) practices offer a solution to these challenges, streamlining the development process and enhancing the reliability of deployments. In this blog, we explore the nuances of CI/CD, examining its significance, and understanding how it can be implemented for full-stack applications.
Understanding the Core Concepts of CI/CD
Continuous Integration (CI) is a development practice where developers regularly integrate their code changes into a shared repository. This integration occurs frequently, often multiple times a day. With each integration, automated tests are run to detect integration errors, ensuring that new changes do not break existing functionality. Continuous Deployment (CD) builds upon the CI process. It involves automatically deploying code changes to production or staging environments after successful testing. The entire deployment process, from code integration to deployment, is automated, minimizing human intervention. CI/CD practices emphasize automation, collaboration, and rapid feedback loops, enabling development teams to deliver high-quality software with confidence.
The Significance of CI/CD for Full Stack Apps
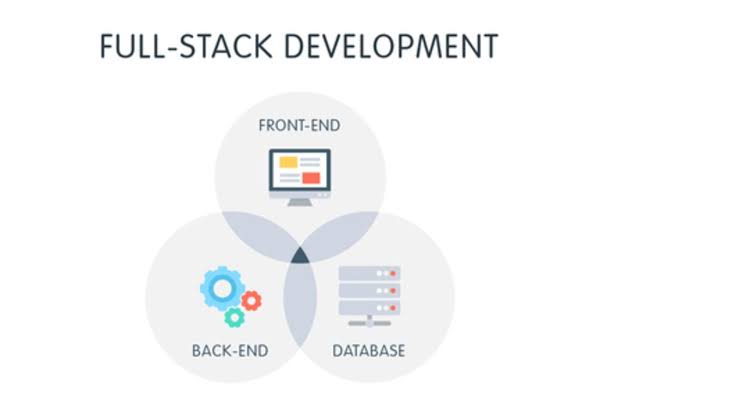
Full-stack applications, with their diverse technologies, components, and dependencies, require meticulous attention to integration and deployment. Here are the key reasons why CI/CD is indispensable for full-stack apps:
Consistency and Compatibility: Full-stack applications often consist of various technologies and frameworks for the front-end and back-end. CI/CD ensures that code changes in both components are tested and integrated consistently, promoting compatibility and smooth interaction between different layers of the application.
Accelerated Development Cycles: By automating the testing and deployment processes, CI/CD accelerates development cycles. Developers can focus on writing code and implementing new features, confident that automated tools handle testing and deployment tasks efficiently. This agility is crucial in today’s fast-paced development environment.
Risk Mitigation: Automated testing and deployments significantly reduce the risk of human errors. Manual deployments are prone to mistakes, which can lead to application downtime or functionality issues. Automated tests catch errors early in the development process, ensuring that only stable and reliable code reaches the production environment.
Scalability and Manageability: Full-stack applications, especially those experiencing rapid growth, require scalable development and deployment processes. Manual deployments become impractical as the complexity of the application increases. CI/CD scales effortlessly, accommodating the demands of growing applications while ensuring efficient management of deployment pipelines.
Enhanced Collaboration and Teamwork: CI/CD fosters a collaborative environment among developers. Continuous integration encourages frequent code integration, allowing teams to identify and resolve conflicts swiftly. Collaboration becomes seamless, leading to better teamwork and knowledge sharing among team members.
Implementing CI/CD for Full Stack Apps: A Step-by-Step Guide
Setting up CI/CD for full-stack applications involves several steps, each tailored to the specific requirements of the project. Let’s explore a detailed guide for implementing CI/CD for a typical full-stack application built with a React front-end and a Node.js back-end.
Step 1: Version Control and Collaboration
Initiate the CI/CD journey by utilizing a robust version control system like Git. Git enables developers to track changes, collaborate efficiently, and maintain a comprehensive history of the codebase. Hosting platforms such as GitHub, GitLab, or Bitbucket provide collaborative environments, facilitating seamless cooperation among team members.
Step 2: Automated Testing for Front-End and Back-End Components
Automated testing is the foundation of CI/CD. For full-stack applications, it is essential to write comprehensive tests for both the front-end and back-end components. Let’s consider an example of writing tests for a React front-end and a Node.js back-end.
Front-End Testing (React)
javascript
// front-end/src/App.test.js
import { render, screen } from ‘@testing-library/react’;
import App from ‘./App’;
test(‘renders app component’, () => {
render(<App />);
const linkElement = screen.getByText(/Hello, World/i);
expect(linkElement).toBeInTheDocument();
});
Back-End Testing (Node.js with Jest):
Back-End Testing
javascript
// back-end/__tests__/app.test.js
const request = require(‘supertest’);
const app = require(‘../app’);
test(‘GET /api/data’, async () => {
await request(app)
.get(‘/api/data’)
.expect(‘Content-Type’, /json/)
.expect(200)
.then(response => {
expect(response.body.data).toBe(‘Hello, World’);
});
});
These tests ensure that both the front-end and back-end components function as expected.
Step 3: CI Configuration
For this example, we will use GitHub Actions as the CI tool. Create a .github/workflows/ci.yml file in your repository to define the CI workflow.
yaml
name: CI
on:
push:
branches:
– main
jobs:
build:
runs-on: ubuntu-latest
steps:
– name: Check out code
uses: actions/checkout@v2
– name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: 14
– name: Install dependencies
run: npm install
– name: Run front-end tests
run: npm test
– name: Run back-end tests
run: npm run test:backend
In this configuration, the workflow runs tests for both the front-end and back-end components upon every push to the main branch.
Step 4: CD Configuration
For Continuous Deployment, deploy the application using a platform like Heroku. Begin by creating a Profile in your back-end directory to specify the command to start the Node.js server:
makefile
web: node app.js
Next, generate a heroku.yml file in your repository root:
yaml
Copy code
build:
docker:
web: Dockerfile
run:
web: npm start
The heroku.yml file defines the build and run configurations for the Heroku deployment.
Create a GitHub Action for Continuous Deployment in the .github/workflows/cd.yml file:
yaml
name: CD
on:
push:
branches:
– main
jobs:
deploy:
runs-on: ubuntu-latest
steps:
– name: Check out code
uses: actions/checkout@v2
– name: Login to Heroku
run: heroku container:login
– name: Build and push Docker container
run: heroku container:push web -a your-app-name
– name: Release app on Heroku
run: heroku container:release web -a your-app-name
In this configuration, the workflow runs upon every push to the main branch, deploying the application to Heroku after successful tests.
Conclusion
Continuous Integration and Continuous Deployment (CI/CD) practices are indispensable for the efficient development and deployment of full-stack applications. By automating testing and deployment processes, CI/CD ensures consistency, accelerates development cycles, reduces risks, and enhances collaboration among development teams. The step-by-step guide provided in this exploration offers a comprehensive overview of implementing CI/CD for a typical full-stack application, empowering developers to navigate the complexities of modern software development with confidence. Embrace CI/CD, and watch your full-stack applications thrive in the competitive and ever-changing digital landscape.
Add comment