Debugging is the lifeblood of software development, an intricate dance between logic and error, where developers unravel complex issues to create seamless applications. In the vast landscape of full-stack development, mastering the art of debugging is not just a skill but it is a necessity. This comprehensive guide delves deep into the world of debugging, exploring fundamental techniques, advanced strategies, and powerful tools that empower full-stack developers to diagnose and fix issues effectively. By understanding these nuances, you’ll not only be equipped to resolve bugs but also elevate your problem-solving skills to the next level.
The Importance of Debugging
Debugging is the essence of software development; it’s the process of finding and resolving defects, enhancing the quality and reliability of your code. Here’s why debugging is paramount in the software development process:
-
Faster Issue Resolution
Swift identification and resolution of bugs lead to faster development cycles. The quicker developers can identify, diagnose, and fix issues, the more efficiently they can deliver high-quality software.
-
Enhanced Code Quality
Effective debugging results in cleaner, more efficient code. Developers gain a deeper understanding of their codebase, leading to improved logic, optimised algorithms, and enhanced overall software quality.
-
Improved Problem-Solving Skills
Debugging challenges developers’ problem-solving abilities. It encourages analytical thinking, logical reasoning, and creativity, transforming developers into proficient troubleshooters.
-
Facilitates Collaborative Development
Clear communication of issues and solutions within a development team fosters collaboration. Debugging techniques enable effective communication, ensuring seamless cooperation among team members.
Basic Debugging Strategies
-
Print Statements
Print statements are the simplest yet most effective debugging tools. By strategically placing print statements in your code, you can observe the flow of execution and the values of variables in real-time. For example, in JavaScript:
javascript
function calculateTotal(price, quantity) {
console.log(‘Calculating total…’);
console.log(‘Price:’, price);
console.log(‘Quantity:’, quantity);
const total = price * quantity;
console.log(‘Total:’, total);
return total;
}
In this example, the console.log() statements provide insights into the variables price, quantity, and total, helping developers understand the function’s behavior during execution.
Using Breakpoints
Modern integrated development environments (IDEs) allow developers to set breakpoints. When the code execution reaches a breakpoint, it pauses, allowing developers to inspect variables, step through the code, and identify issues. For instance, in JavaScript:
javascript
function processOrder(order)
{
debugger; // Set a breakpoint
// Process the order
}
By setting a breakpoint with the debugger; statement, developers can interactively examine the order variable and other aspects of the code at that specific point in the execution flow.
Explaining these basic strategies in detail provides developers with a solid foundation for understanding how to observe and manipulate their code during execution, laying the groundwork for more advanced debugging techniques.
Advanced Debugging Techniques
Interactive Debuggers
Interactive debuggers, such as Python’s pdb and JavaScript’s node-inspect, provide developers with a powerful command-line interface for debugging. These tools allow developers to set breakpoints, step through code, inspect variables, and even modify variables in real-time. For example, in Python:
python
# Python PDB Example
import pdb
def calculate_total(price, quantity):
pdb.set_trace() # Set a breakpoint
total = price * quantity
return total
In this example, pdb.set_trace() sets a breakpoint, enabling developers to interactively debug the calculate_total function, gaining insights into variable values and code execution flow.
Logging Frameworks
Logging frameworks, such as Java’s log4j, Node.js’ winston, and Python’s built-in logging, enable developers to create detailed logs. These logs capture critical events, variable values, and function calls, providing invaluable insights into the application’s behaviour. For example, in Node.js with Winston:
javascript
// Node.js Winston Logging Example
const winston = require(‘winston’);
const logger = winston.createLogger({
level: ‘debug’,
format: winston.format.json(),
transports: [
new winston.transports.Console({ format: winston.format.simple() })
]
});
function processOrder(order) {
logger.debug(‘Processing order:’, order);
// Process the order
}
In this above code, the logger.debug() statement captures details about the order being processed, facilitating comprehensive logging for effective debugging and analysis.
Explaining these advanced techniques in detail equips developers with the knowledge and understanding necessary to apply these methods effectively in real-world scenarios, ensuring efficient issue diagnosis and resolution.
Debugging Tools for Full Stack Development
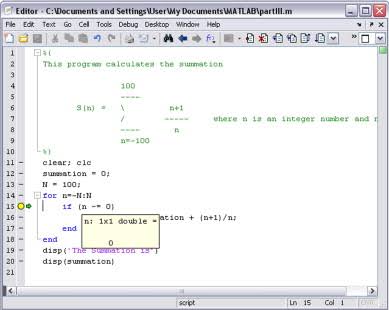
Chrome DevTools
Chrome DevTools is a comprehensive set of web development and debugging tools integrated into the Chrome browser. It provides real-time inspection of web pages, JavaScript debugging, performance profiling, and network activity analysis. For front-end and Node.js developers, Chrome DevTools is an indispensable asset for debugging and optimising applications.
Visual Studio Code Debugger
Visual Studio Code (VS Code) boasts a powerful built-in debugger supporting multiple programming languages and frameworks. Its intuitive interface allows developers to set breakpoints, evaluate expressions, inspect variables, and even debug applications running in remote environments. VS Code’s debugger enhances the debugging experience, enabling developers to diagnose and fix issues efficiently.
Postman
Postman, primarily known as an API development tool, serves as an excellent API debugging companion. It allows developers to create and execute HTTP requests, view detailed responses, inspect headers, and validate API endpoints. Postman’s user-friendly interface simplifies API testing and debugging, making it an invaluable tool for full-stack developers working with APIs.
Advanced Debugging Scenarios
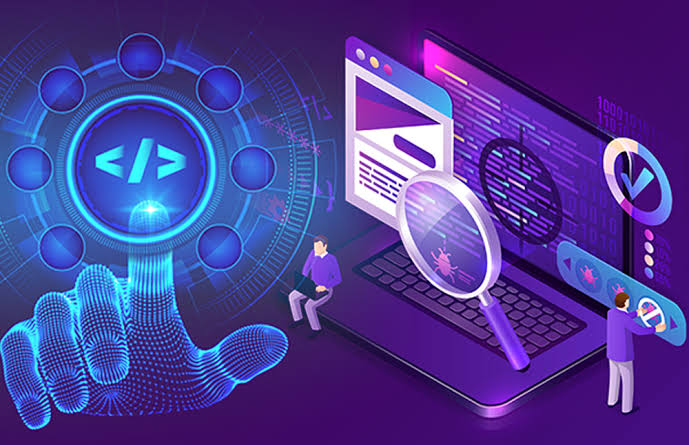
Remote Debugging
Remote debugging is essential for diagnosing issues that occur in production or remote environments. For JavaScript applications, tools like node-inspect provide remote debugging capabilities, enabling developers to connect to a running application for real-time issue diagnosis. Remote debugging techniques empower developers to address issues efficiently, even in challenging, real-world scenarios.
javascript
// Remote Debugging in Node.js
const inspector = require(‘inspector’);
inspector.open(9229, ‘0.0.0.0’, true);
In this example, the Node.js inspector module opens a remote debugging session on port 9229, allowing developers to connect and debug the application remotely.
Memory Profiling
Memory leaks and inefficient memory usage can lead to performance degradation in applications. Tools like Chrome DevTools’ Memory panel allow developers to profile memory usage, identify memory leaks, and optimise applications for superior performance. By analysing memory usage patterns and identifying potential leaks, developers can enhance the application’s efficiency and responsiveness, ensuring a seamless user experience.
javascript
// Memory Profiling in JavaScript
function createHeavyObject() {
const obj = [];
for (let i = 0; i < 1000000; i++) {
obj.push({ key: ‘value’ });
}
return obj;
}
const heavyObject = createHeavyObject();
In this code, the createHeavyObject() function creates an array with a million objects, potentially causing a memory leak. Memory profiling tools can help developers identify and optimise such memory-intensive operations.
Conclusion
In conclusion, debugging is not just a technical skill—it’s an art. It requires patience, curiosity, and a deep understanding of the code. By mastering a combination of basic strategies, advanced techniques, and powerful debugging tools, developers can navigate the complexities of full-stack development with confidence. Debugging transforms developers into detectives, uncovering hidden issues and ensuring the reliability and performance of their software creations.Thus, developers need to step up to the challenges, hone the debugging skills, and let the expertise illuminate the path to bug-free, high-quality software solutions.
Add comment