In the ever-evolving digital landscape, the bedrock of web application security lies in the seamless orchestration of authentication and authorization. These twin pillars not only validate the identity of users but also govern their access to critical resources. As we look into the complex web of security protocols, this blog will help us understand the state of two formidable technologies – JSON Web Tokens (JWT) and OAuth. Beyond mere introductions, we will also dive deep into their mechanisms, providing not just code examples but a profound understanding of their roles in fortifying the security infrastructure of web applications.
What is Authentication?
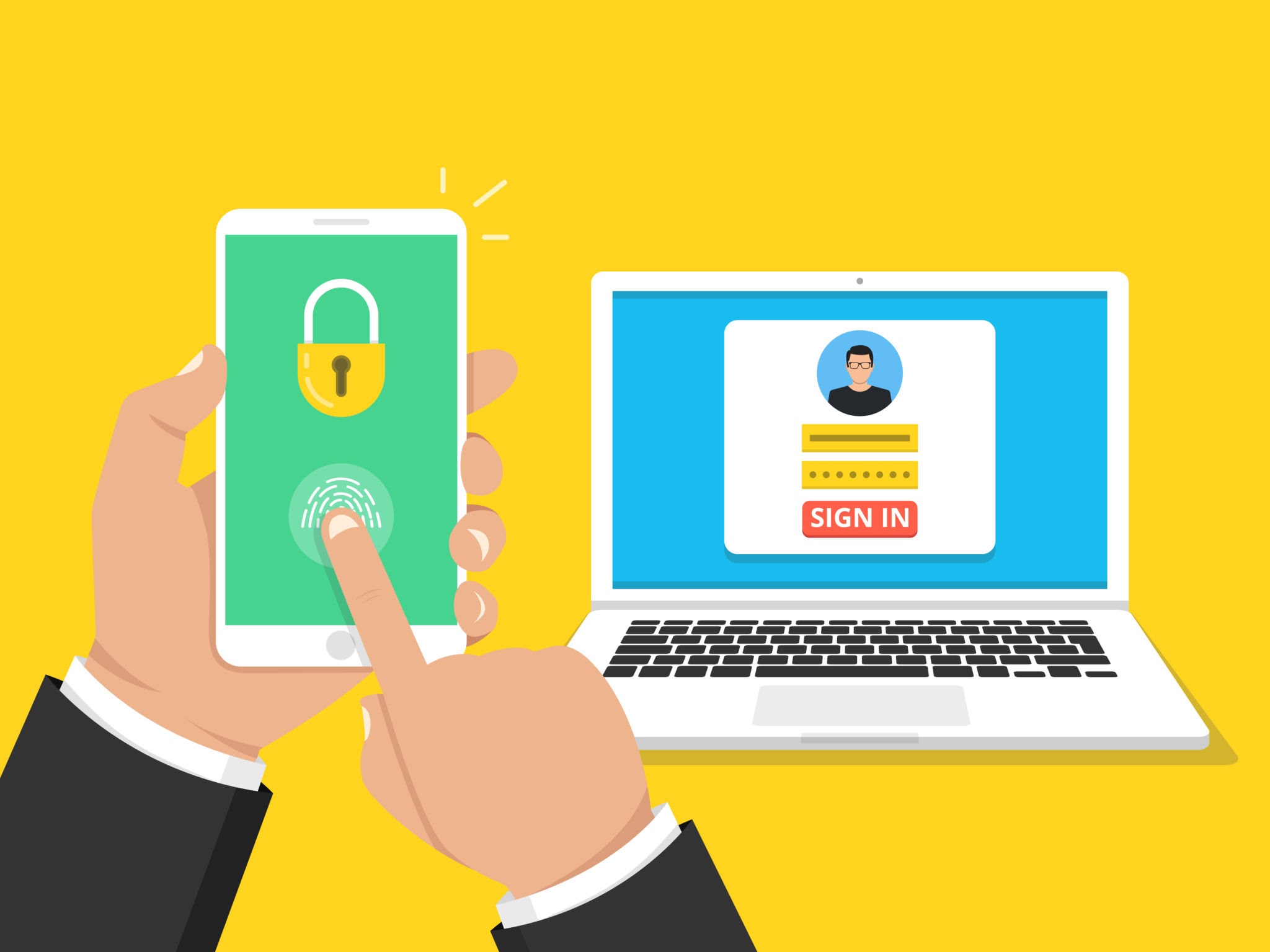
Authentication, once a realm dominated by traditional username-password combinations, has undergone a metamorphosis. The rise of token-based authentication has become a hallmark of modern security practices.
The Evolution of Authentication Methods
The conventional approach of username and password is now augmented by token-based authentication. Tokens, being self-contained units, revolutionize the user verification process, reducing the dependency on repeated database queries during user interactions.
The Essence of Token-Based Authentication with JWT
JSON Web Tokens (JWTs) stand out as a beacon in the realm of token-based authentication. Comprising a header, payload, and signature, JWTs offer a compact yet robust solution for transmitting crucial user information.
How to Generate a JWT in Node.js
javascript
const jwt = require(‘jsonwebtoken’);
const secretKey = ‘your-secret-key’;
const user = { id: 123, username: ‘example_user’ };
const token = jwt.sign(user, secretKey, { expiresIn: ‘1h’ });
console.log(‘Generated JWT:’, token);
How to Verify a JWT in Node.js
javascript
try {
const decodedUser = jwt.verify(token, secretKey);
console.log(‘Decoded User:’, decodedUser);
} catch (error) {
console.error(‘Token verification failed:’, error.message);
}
These examples not only generate and verify JWTs but symbolize the transition from traditional to token-based authentication.
Introduction to JWT
Unraveling the Layers of JWT
When we look deeply into a certain aspect, it is bound to reveal something interesting. In this case, the JWT reveals a versatile structure. The header defines the token type and signing algorithm, while the payload encapsulates user claims, creating a self-contained unit of information.
Unlocking the Benefits of JWT
Beyond their compact format, JWTs boast self-containment, reducing the need for frequent database queries. The flexibility of JWTs positions them as a versatile solution applicable across a myriad of scenarios.
What is Authorization?
As a natural companion to authentication, authorization steps into the limelight, delineating user permissions within a system. Role-Based Access Control (RBAC) serves as a guiding principle, associating roles with specific actions and resources.
Navigating Authorization with Role-Based Access Control (RBAC)
RBAC simplifies authorization by assigning roles to users. Each role carries specific permissions, streamlining the process of determining who can access what.
An Overview of OAuth
The Power of OAuth in Authorization
OAuth, standing as an open standard for access delegation, facilitates secure third-party access to resources without exposing user credentials. A nuanced understanding of its roles and flows is essential for effective implementation.
Revisiting OAuth Roles
Resource Owner: The user authorizing an application to access their resources.
Client: The application seeking access to user resources.
Authorization Server: Validates the user’s identity and issues access tokens.
Resource Server: Hosts protected resources and handles requests based on access tokens.
A Closer Look at OAuth Flows
Diving into OAuth flows provides insight into choosing the right approach based on the application’s architecture and requirements.
Implementing OAuth2.0 Implicit Flow
javascript
const express = require(‘express’);
const bodyParser = require(‘body-parser’);
const OAuth2 = require(‘oauth2’);
const app = express();
app.use(bodyParser.urlencoded({ extended: true }));
const clientId = ‘your-client-id’;
const redirectUri = ‘your-redirect-uri’;
const oauth2Client = new OAuth2(clientId, null, ‘https://api.example.com’, ‘/oauth/token’, ‘/oauth/authorize’);
app.get(‘/login’, (req, res) => {
const authorizationUrl = oauth2Client.implicit.authorizeURL({
redirect_uri: redirectUri,
scope: ‘scope1 scope2’,
});
res.redirect(authorizationUrl);
});
app.get(‘/callback’, (req, res) => {
const { access_token } = req.query;
console.log(‘Access Token:’, access_token);
res.send(‘Authentication successful!’);
});
const port = 3000;
app.listen(port, () => {
console.log(`Server running on http://localhost:${port}`);
});
This example demonstrates the OAuth2.0 Implicit Flow using an Express server, providing a practical illustration of OAuth in action.
Combining JWT and OAuth for Secure Authentication and Authorization
The combination of JWT and OAuth transcends individual capabilities, creating a robust framework for authentication and authorization. Picture a scenario where a user logs in using their Google or Facebook account. OAuth seamlessly handles the authentication, and the resultant access token, now a JWT, encapsulates not only the user’s identity but also pertinent permissions.
How is Social Media Authentication Carried Out?
Consider a scenario where a web application allows users to sign in using their Google or Facebook accounts. OAuth handles the authentication, and the resultant access token can be a JWT, carrying necessary user information.
Best Practices for Secure Authentication and Authorization
Ensuring the security of authentication and authorization systems requires adopting best practices.
Regularly Update Secrets and Tokens
Frequent rotation of secret keys and token refreshment reduces the risk of compromise.
Token Expiration and Refresh Mechanisms
Setting reasonable token expiration times and implementing refresh mechanisms ensures continuous security.
Monitoring and Logging
Vigilant monitoring and logging play a pivotal role in identifying and responding to suspicious activities promptly.
Password Security and User Sessions
Securely storing passwords with strong hashing algorithms and managing user sessions enhance overall system security.
Future Trends and Considerations
Biometric Authentication
As technology advances, the integration of biometric authentication, such as fingerprint or facial recognition, is becoming more prevalent, enhancing user authentication.
Decentralized Identity
The emergence of decentralized identity solutions, like blockchain-based systems, offers new paradigms for secure and privacy-enhanced authentication.
Conclusion
In the vast universe of web application security, the symbiotic relationship between authentication and authorization finds its zenith in the amalgamation of JWT and OAuth. As we traverse this digital odyssey, it becomes evident that security is not a static destination but a dynamic journey. Each line of code, each security protocol, and each technological advancement contribute to the fortification of our digital ecosystem. As the horizon of possibilities expands, the commitment to security remains unwavering, ensuring a resilient and robust future for web applications
Add comment